Migrating to NextJS and Deploying to Vercel
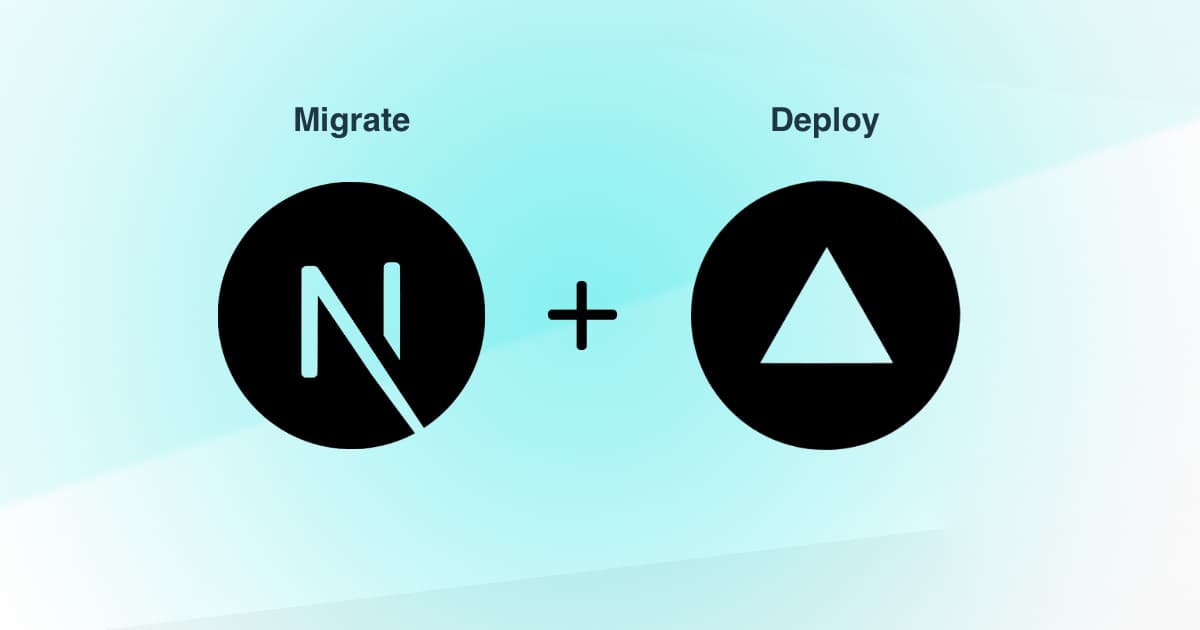
Introduction
In my previous post, I went over the details of how I built this blog using Lovable, Cursor and Claude. This is a second part to that post as this covers the deployment process.
I wanted to create an SEO friendly blog website and I read a research post from Vercel that stated that new AI search crawlers like Perplexity and Anthropic were unable to render JS pages. I also learnt that there is a concept of Server Side Rendering that allows the webpage to be rendered on the server before being sent to the client.
All this to say that I had to do two things:
- Migrate the website to use NextJS + React instead of the Vite + React that it was currently using.
- Since I was hosting the website on Vercel, NextJS felt like a good fit since they are the creators of it.
Migrating to NextJS
Migrating a project from one framework to another is a challenge whether you are doing something at scale or even for a small project like mine. Since I wanted Cursor to assist me in this endeavor, I started asking questions like:
Can you give me a path to server side rendering atleast for the blog post pages? Do not implement any code yet
It responded with:
Here's a strategic path to implement Server-Side Rendering (SSR) for blog posts:
Migration Options:
Next.js (Recommended): Built-in SSR, great React support
Remix: Full-stack framework with SSR
Vite SSR: More complex, manual setup
Next.js Migration Path:
Keep existing components and logic
Convert pages to Next.js format
Implement getStaticProps for blog posts
Setup dynamic routes
Configure Supabase for SSR
Key Changes Needed:
Move blog posts to /pages/blog/[slug].tsx
Implement static generation for posts
Update meta tags for SEO
Adjust data fetching patterns
Handle image optimization
Benefits:
Better SEO
Faster initial page load
Improved Core Web Vitals
Better social media previews
Reduced client-side JavaScript
Would you like me to:
Show the Next.js implementation plan?
Explain the specific changes needed?
Create a migration guide?
Since the recommended option was to use NextJS I then asked:
Walk me through it step by step
It then started giving me instructions on how to create a new NextJS project and install depenencies. I had to copy the step output from my old project to the new project’s chat input and keep doing this until all the code was migrated.
I won’t go into every step here but I did observe a few things:
- It can completely forget the existence of certain features/pages. It forgot about the About page I had as well as the editor page I had. It also forgot about the dark mode toggle. It instead setup a three way toggle of light, dark and system.
- It completely changed the design language of the website. It removed some nice color highlighting that I had done for buttons in my post editor page.
It added a few things that are worth mentioning:
Server Side Rendering: Page revalidation
It added a Revalidation Token to allow only authorized users to revalidate the page in NextJS. To do the revalidation, it added a new function in Supabase:
-- This function will be triggered when posts are updated
create or replace function handle_post_update()
returns trigger as $$
begin
-- Make HTTP request to revalidation endpoint
select http.post(
'https://talesofrm.dev/api/revalidate',
'{"path": "/blog/' || NEW.slug || '"}',
'{
"Content-Type": "application/json",
"Authorization": "Bearer your_revalidation_token_here"
}'
);
return NEW;
end;
$$ language plpgsql security definer;
and then a trigger needed to be created:
create trigger on_post_update
after update or insert
on posts
for each row
execute procedure handle_post_update();
So the flow from my understanding would be, when a post update occurs from my editor view, the updated post would be written to the posts database. After the write is completed, a trigger called on_post_update
triggers the function handle_post_update
. This function then revalidates the post URL to provide the latest update to all readers.
Deploying to Vercel
I originally had deployed the old version of my website to Vercel and purchased a domain with them for this site. I had to figure out how I could deploy the new NextJS repo to Vercel and point the domain to the new project. Again I asked in the chat for some options and the best one I got is to create a new repository in GitHub and a new project in Vercel that points to this repo. Post-deployment, the Domain can be disassociated from the old project to the new one.
The steps to deploy to Vercel are pretty easy:
- Sign up for an account.
- Login to your account and connect your Github profile to it.
- Click on Add new -> Project and you should see an option to import a Git repository.
- Upload your code from Cursor to Github by creating a new repo in Github and pushing your code to it.
- In Vercel, choose the repo you want and then set any environment variables that you had in your local environment. In my case, since I was connecting to Supabase, I had a few environment variables related to that.
- Hit Deploy and wait for it to finish deploying. In case you see errors, you can come back to Cursor and paste the logs from the deployment to debug any issues.
- Once the deployment is successful you should see a link (should end in .vercel.app) that will point to your deployed application.
- If you have any domain names purchased with Vercel, you can associate it to the project.
That’s it! Thanks for reading. I like to log my dev notes in this format. I’m happy to take feedback through my socials which can be found on the About page.